Introduction: What is React?
React, is an open-source JavaScript library used for building user interfaces or UI components. It was developed by Facebook and is maintained by Facebook and a community of individual developers and companies. React can be used to create single-page applications and mobile applications. It aims to be both efficient and flexible, designed with a component-based architecture, allowing developers to build complex UIs from simple, reusable pieces of code called components.
React implements a virtual DOM, which is a lightweight copy of the actual DOM. This feature increases application performance because React compares the current and the new virtual DOMs before each update and applies only the differences to the actual DOM, instead of updating the whole DOM.
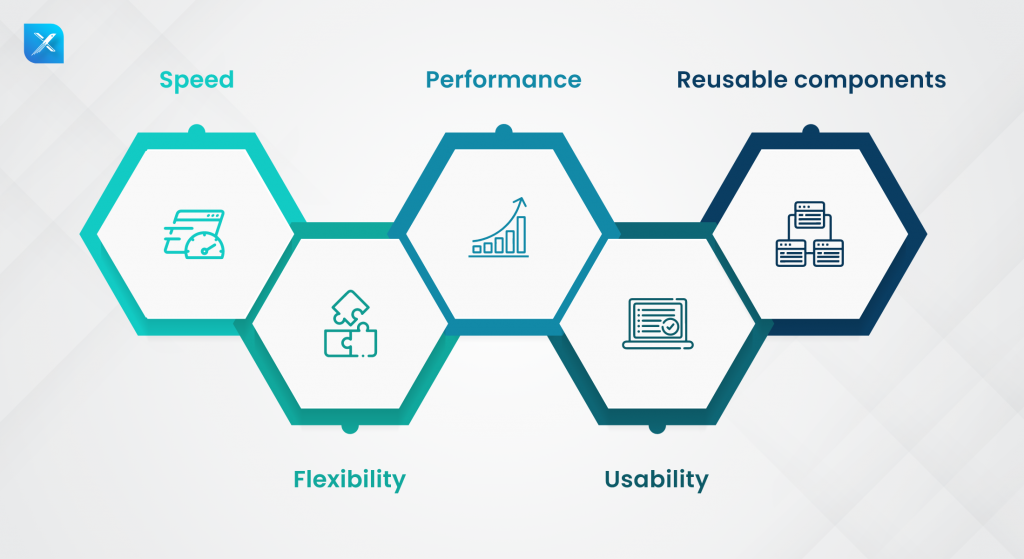
Single-Page Applications (SPAs)
Single-page applications (SPAs) are web applications that load a single HTML page and dynamically update that page as the user interacts with the app. This kind of application is particularly suitable when developed with React.
In a traditional multi-page application, every time a user navigates to a new page or requests data, the browser makes a new request to the server and reloads the whole page with the new data. This can result in slow page loads and a disjointed user experience.
React, when used to create SPAs, instead only updates the necessary parts of the web page. When a user navigates to a new page or requests new data, React can use JavaScript to fetch the new data and update only the components that need to change. This results in a much smoother user experience, similar to a desktop application.
React Router is a popular library used in conjunction with React to implement navigation in SPAs. It allows you to sync your UI with the URL, handling different URL paths, and dynamically rendering different components based on the current path.
An SPA developed with React, therefore, is fast and efficient, providing an excellent user experience with seamless navigation and without the need for constant server requests and full page reloads.
Reusable Components
React's reusable components are one of its most powerful features, providing a range of benefits that can significantly enhance the development process. Here are a few ways in which reusable components are helpful for development:
- Code Reusability and Efficiency: Components are like custom, reusable pieces of code. If a particular element is used in multiple places in the application, a component can be created once and then reused, eliminating the need to write new code each time.
- Consistency Across the App: Reusing components helps maintain a consistent look and feel across the application, as the same pieces of UI are used in multiple places.
- Easier Maintenance and Updates: When a component needs to be updated or fixed, the changes will propagate to every instance of that component. This makes maintaining and updating the codebase much easier and less error-prone.
- Separation of Concerns: Each component in React encapsulates its own structure (markup), style (CSS), and behavior (JavaScript). This separation of concerns makes the code easier to understand and manage.
- Faster Development: Components can act like building blocks. Developers can compose them in various ways to quickly build complex UIs. The more components a team has at its disposal, the quicker new pages can be put together.
- Easier Testing: Because components are isolated and reusable, they can be unit tested in isolation. This leads to more robust code and easier debugging.
To illustrate, consider a simple example such as a Button component. If you have a standard style for buttons across your application, instead of defining the button's style and behavior each time you use it, you can define a Button component once and reuse it throughout your application. Any changes to the Button component (such as a color change or a hover effect) would then automatically apply wherever the Button component is used.
Virtual DOM
What is the Virtual DOM?
The Virtual DOM is a programming concept where an ideal or "virtual" representation of a UI is kept in memory and synced with the "real" DOM by a library such as ReactDOM. It's a step that occurs between the rendering of a component and the display in the HTML DOM.
How Does the Virtual DOM Work?
When a component's state changes, a new virtual DOM representation of the user interface is created. React then compares this newly created virtual DOM with the old one (a process known as "diffing") and calculates the most efficient way to update the real DOM to match the new virtual one.
This process involves several steps:
- Whenever any underlying component data changes, the entire UI is re-rendered in Virtual DOM representation.
- The difference between the previous Virtual DOM representation and the new one is calculated.
- The real DOM is updated with what was actually changed. This is called reconciliation.
React uses a smart diffing algorithm to minimize the number of operations it needs to perform to update the real DOM.
Why is the Virtual DOM Beneficial?
- Improved Performance: Since the real DOM's manipulations are costly and the virtual DOM is much faster, the diffing process results in a significant performance boost. Only the components that actually need to update get re-rendered, rather than the whole application.
- Code Abstraction: The Virtual DOM provides a simple programming model to the developer. You just need to update the component state, and React will automatically update the UI to match this state.
- Efficiency: By minimizing direct manipulation of the DOM and batching multiple changes together, React reduces the load on the browser and enhances efficiency.
So, the Virtual DOM allows React to be fast by avoiding direct manipulation of the real DOM and by updating only the parts of the real DOM that need to change.
Rich JavaScript Library
React isn't an opinionated framework. It doesn't dictate a specific way of doing things. Instead, it provides a rich JavaScript library that allows developers to choose their paths, making it a flexible and customizable tool.
Strong Community
React has an enormous and vibrant community of developers. This guarantees a wealth of learning resources, regular updates, and an abundance of third-party libraries.
React in the Real World
Companies such as Facebook, Instagram, and WhatsApp utilize React in their applications, demonstrating its reliability and scalability.
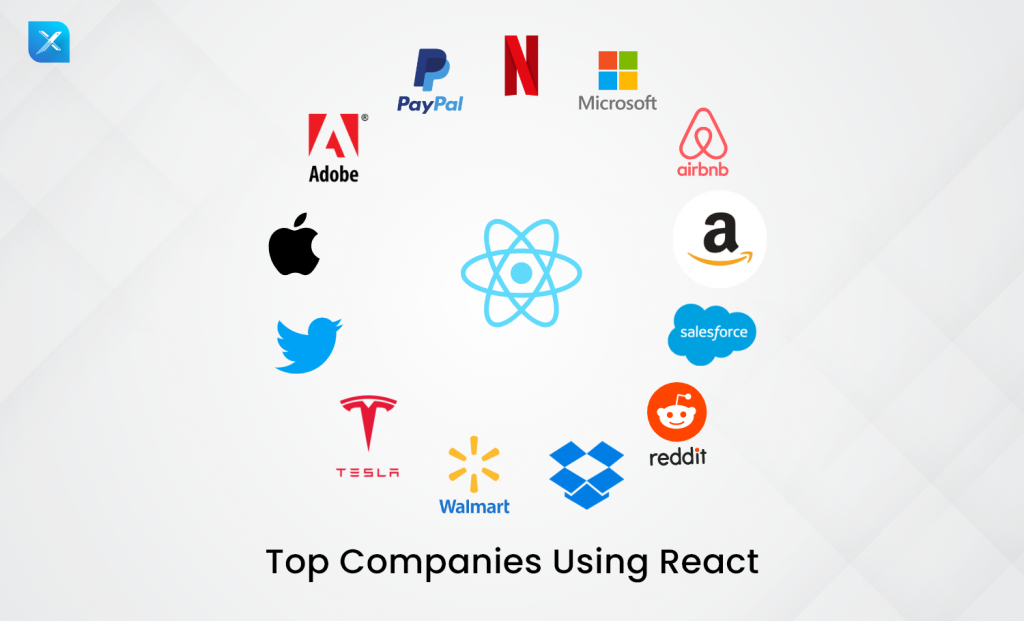
4 React Facts
- React is the most popular JavaScript framework. According to Stack Overflow's 2022 Developer Survey, React is the most popular JavaScript framework, with over 70% of developers using it.
- React is used by some of the biggest tech companies. According to Career Kaarma, Some of the biggest tech companies in the world, such as Facebook, Instagram, and Netflix, use React. This is a testament to React's ability to build large, complex applications.
- React is fast. React uses a virtual DOM, which makes it very fast. The virtual DOM is a lightweight representation of the real DOM, which means that React only updates the real DOM when necessary. This makes React applications feel very responsive.
- React is scalable. React is very scalable. This is because React components are small and reusable, which makes it easy to add new features or change existing features without affecting the rest of the application.
10 Frequently Asked Questions About React
Q: What is React?
A: React is a powerful JavaScript library for building user interfaces, especially single-page applications. It allows developers to create reusable UI components.
Q: Who developed React and why?
A: React was developed by Facebook. It was designed to improve efficiency and maintainability by allowing developers to create reusable UI components that manage their own state.
Q: What are the main advantages of using React?
A: React offers several advantages, including the ability to create reusable components, a virtual DOM that improves app performance, a rich JavaScript library, a strong community, and a lower learning curve compared to some other libraries and frameworks.
Q: What is JSX and why is it important in React?
A: JSX stands for JavaScript XML. It allows developers to write HTML in React, thereby making the code more readable and easier to write.
Q: Can I use React for mobile application development?
A: Yes, React Native, a derivative of React, is used for mobile application development. It allows developers to write native mobile applications in JavaScript using the same design as React.
Q: How does React handle data flow?
A: React follows a unidirectional data flow or one-way data binding. The model state updates the view but not vice versa.
Q: What is Redux and when should I use it?
A: Redux is a predictable state container designed to help you write JavaScript apps that behave consistently across client, server, and native environments. While Redux is not required to build an application in React, it's extremely useful for handling complex state interactions in larger applications.
Q: How does React improve performance?
A: React improves performance using the "virtual DOM". When the state of an object changes, React just updates that object in the virtual DOM, not the real DOM. It then calculates the best way to make these changes and updates only the required parts of the real DOM.
Q: How can I handle events in React?
A: In React, you can handle events very similarly to how you would in regular DOM. However, in React, you cannot return false to prevent default behavior. Instead, you must call preventDefault.
Q: What is a 'component' in React? A: Components are the building blocks of any React application. A component is a self-contained module that renders some output. A React application usually consists of multiple components that work together while being independent of each other.ns.
Final Thoughts
With its unique set of features, including reusable components, the Virtual DOM, and a rich JavaScript library, React is undoubtedly a robust tool for modern web development. Whether you're a seasoned developer or just starting, React provides a versatile, efficient, and enjoyable development experience.
Are you ready to leverage the power of React in your next web project? Dive into the official React documentation and start your journey today!